Turbocharge Your C++ Quant Trading System: Speed Optimization Secrets
- Bryan Downing
- Feb 13
- 5 min read
In the cutthroat world of quant trading, speed is paramount. A microsecond advantage can translate into millions of dollars in profit. For C++ quant trading systems, optimizing for speed isn't just a good idea – it's a necessity. This article unveils the secrets to squeezing every ounce of performance from your C++ code, giving you a competitive edge in the high-frequency trading arena.
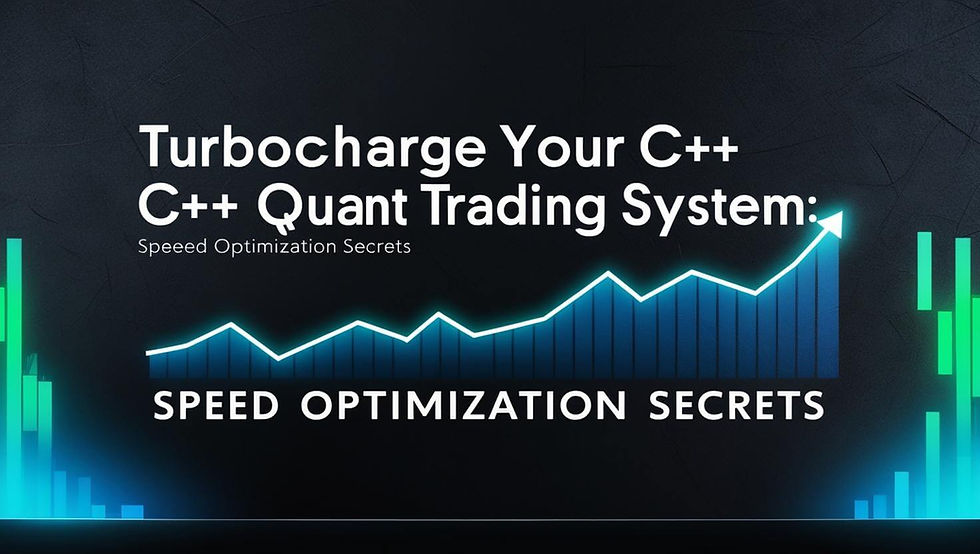
Why Speed Matters in Quant Trading
In today's fast-paced markets, the difference between winning and losing often comes down to milliseconds. Here's why speed is so critical:
Microsecond Advantage: In high-frequency trading (HFT), opportunities can vanish in the blink of an eye. A microsecond advantage can be the difference between capturing a profitable trade and missing it entirely.1
Faster Execution = Higher Returns: Faster execution allows you to capitalize on market movements more quickly, increasing your potential returns.
Competitive Edge: In a highly competitive environment, speed is a key differentiator. Traders with faster systems have a significant advantage over those with slower ones.2
Common C++ Bottlenecks
Several common bottlenecks can slow down C++ quant trading systems:
Memory Allocation and Deallocation: Frequent memory allocations and deallocations can be expensive. Dynamic memory management can introduce significant overhead.3
Loop Optimization and Vectorization: Inefficient loops can significantly impact performance.4 Failing to leverage vectorization can leave substantial performance gains on the table.
Unnecessary Data Copies: Copying large amounts of data can be time-consuming. Minimizing data copies is crucial for performance.
I/O Operations: Disk and network I/O can be slow. Optimizing I/O operations is essential, especially when dealing with large datasets.
Function Call Overhead: Excessive function calls, especially small, frequently called functions, can add up.
Profiling Tools for C++
Before optimizing, you need to identify the bottlenecks in your code. Profiling tools can help you pinpoint the areas that need the most attention:
Valgrind: Valgrind is a powerful suite of tools for memory debugging and profiling.5 It can help you identify memory leaks, memory corruption, and performance issues related to memory usage.
gprof: gprof is a classic profiling tool that can help you identify the functions that are called most frequently.6 This can give you a good starting point for optimization.
VTune Amplifier: Intel VTune Amplifier is a more advanced profiling tool that provides detailed code-level profiling information.7 It can help you identify hotspots in your code and pinpoint the exact lines of code that are causing performance bottlenecks.
Optimization Techniques
Once you've identified the bottlenecks, you can apply various optimization techniques:
Use STL Algorithms: The Standard Template Library (STL) provides a rich set of algorithms that are highly optimized.8 Using STL algorithms can often be much faster than writing your own loops.
Leverage Multi-threading: Modern CPUs have multiple cores.9 Multi-threading allows you to take advantage of these cores by running different parts of your code in parallel.10 This can significantly speed up your system.
Reduce Memory Allocations: Minimize the number of dynamic memory allocations by using memory pools or pre-allocating memory. Memory pools can significantly reduce the overhead associated with memory management.11
Vectorization: Modern CPUs support Single Instruction, Multiple Data (SIMD) instructions, which allow you to perform the same operation on multiple data elements simultaneously.12 Vectorizing your code can significantly improve performance.13
Caching: Caching frequently accessed data can reduce the number of memory accesses, improving performance.14
Code Optimization: Optimize your code for readability and efficiency. Avoid unnecessary computations and use efficient data structures.
Profiling-Guided Optimization: Use profiling tools to guide your optimization efforts. Focus on the areas of your code that are causing the biggest performance bottlenecks.
Fastflow Example
Fastflow is a C++ template library for developing high-performance, concurrent applications.15 It provides a set of primitives for building parallel pipelines and dataflow networks.16 Using Fastflow can be a powerful way to optimize your quant trading system. You can find examples and reports on Fastflow's performance on platforms like GitHub. These resources often demonstrate how Fastflow can be used to significantly improve the speed of various applications, including those relevant to quant trading.
Case Study: Speeding Up Your System
Let's consider a real-world example: optimizing a backtesting loop. Suppose you have a backtesting loop that iterates over historical market data. A common bottleneck in this type of loop is data access. You can optimize this loop by caching the data in memory and using vectorized instructions to perform calculations.
Here's a simplified example (before optimization):
C++
for (int i = 0; i < data.size(); ++i) {
double price = data[i].price;
// ... (perform calculations) ...
}
After optimization (using caching and vectorization - conceptually):
C++
// ... (cache data in memory) ...
for (int i = 0; i < data.size(); i += 4) { // Process 4 elements at a time (vectorization)
__m256d prices = mm256loadu_pd(&data[i].price); // Load 4 prices
// ... (perform vectorized calculations on prices) ...
}
By caching the data and using vectorized instructions, you can significantly speed up the backtesting loop.
Benchmarks and Performance Comparison
After applying optimizations, it's essential to benchmark your code to measure the performance improvements. Use benchmarking tools to compare the performance of your code before and after optimization. This will help you quantify the impact of your optimizations and identify any remaining bottlenecks.
Practical Takeaways and Best Practices
Profile First: Always profile your code before optimizing. Don't make assumptions about where the bottlenecks are.
Focus on Bottlenecks: Concentrate your optimization efforts on the areas of your code that are causing the biggest performance problems.17
Measure Improvements: Use benchmarking tools to measure the impact of your optimizations.
Iterate: Optimization is an iterative process.18 Continue to profile and optimize your code until you've achieved the desired performance.
Maintainability: Balance performance with code maintainability. Don't sacrifice readability and maintainability for minor performance gains.
By following these best practices, you can build a C++ quant trading system that is both fast and efficient, giving you a crucial advantage in the financial markets.
The secrets to optimizing your C++ quant trading system for speed are revealed. Discover how to squeeze every ounce of performance from your code and gain a competitive edge in the high-frequency trading world.
Outline
Why Speed Matters in Quant Trading
Microsecond advantage in the market
Faster execution = higher returns
Competitive edge in high-frequency trading
Common C++ Bottlenecks
Memory allocation and deallocation
Loop optimization and vectorization
Unnecessary data copies
Profiling Tools for C++
Valgrind: memory leaks and performance issues
gprof: function call profiling
VTune Amplifier: code-level profiling
Optimization Techniques
Use STL algorithms for efficient operations
Leverage multi-threading for parallel processing
Reduce memory allocations by using memory pools
Fastflow example on Github report
Case Study: Speeding Up Your System
Real-world example: optimizing a backtesting loop
Benchmarks and performance comparison
Practical takeaways and best practices
Comments