High-Frequency Trading (HFT) demands extreme performance from its software. Every microsecond shaved off execution time can translate into significant profit. In this relentless pursuit of speed, C++ remains the language of choice due to its low-level control and performance characteristics.1 While traditional optimization techniques like C++ template lamdas,loop unrolling and branch prediction are crucial, the power of template lambdas offers a potent tool for further acceleration, particularly in data-intensive HFT applications.
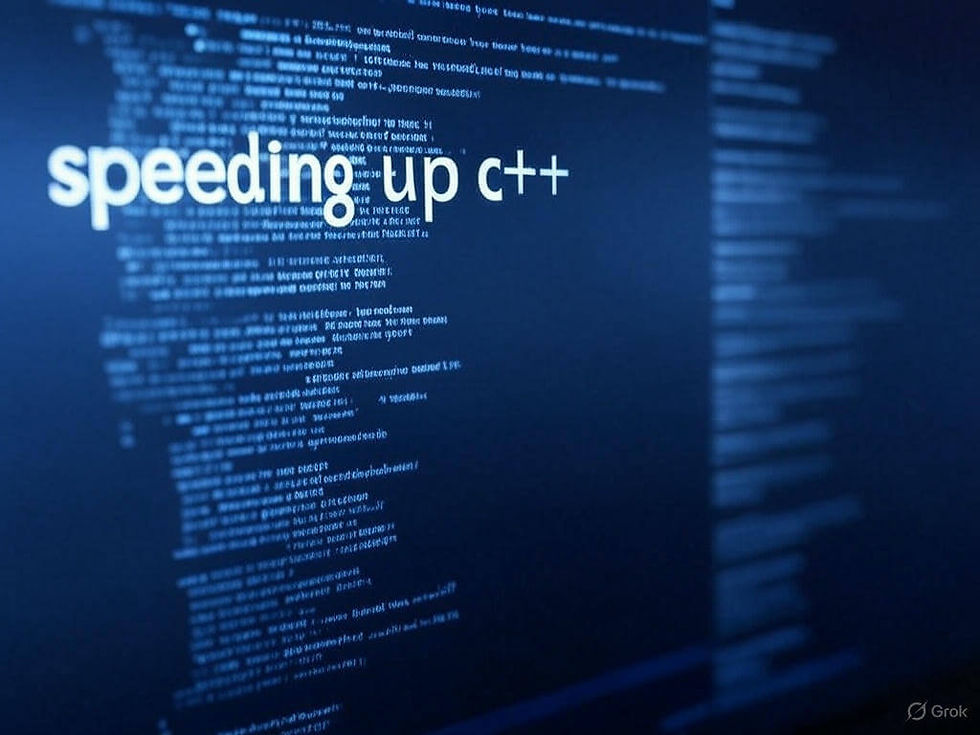
Template lambdas, introduced in C++14 and enhanced in subsequent standards, combine the flexibility of lambdas with the compile-time optimization capabilities of templates. This synergy allows for the generation of highly specialized code, tailored to specific data types and operations, minimizing runtime overhead.
Understanding the Fundamentals
Before diving into HFT-specific applications, let's recap the basics of template lambdas. A regular lambda expression defines an anonymous function object.2 A template lambda, on the other hand, allows you to parameterize the lambda with template parameters, enabling the lambda to work with a variety of types.
C++
auto generic_operation = []<typename T>(T a, T b) {
return a * b + a;
};
int result_int = generic_operation(5, 10);
double result_double = generic_operation(3.14, 2.71);
In this example, generic_operation is a template lambda that can operate on any type T for which multiplication and addition are defined. The compiler generates specialized versions of the lambda for int and double at compile time, eliminating the runtime overhead associated with generic function calls.
Applying Template Lambdas in HFT
HFT algorithms often involve repetitive operations on large datasets, such as order book processing, market data analysis, and risk calculations. Template lambdas can significantly accelerate these operations by:
Eliminating Virtual Function Calls: In object-oriented designs, virtual function calls can introduce performance bottlenecks. Template lambdas, when used in conjunction with compile-time polymorphism (CRTP, for example), can replace virtual calls with statically dispatched function calls, reducing overhead.
Specializing for Specific Data Types: HFT systems often deal with fixed-precision numbers, such as int64_t for price and quantity. Template lambdas allow you to write generic algorithms that are specialized for these specific types, maximizing performance.
Inlining Complex Operations: Template lambdas can be inlined by the compiler, even for complex operations, further reducing function call overhead. This is especially beneficial for short, frequently executed operations.
Meta-Programming for Custom Optimizations: Template metaprogramming, combined with template lambdas, allows you to generate highly optimized code based on compile-time information. For example, you can generate specialized algorithms for different order book depths or market conditions.
Practical Examples
Consider an HFT application that needs to calculate the weighted average price of a portion of the order book.
C++
template <typename PriceType, typename QuantityType, typename Operation>
PriceType calculate_weighted_average(const std::vector<std::pair<PriceType, QuantityType>>& orders, size_t num_orders, Operation op) {
PriceType weighted_sum = 0;
QuantityType total_quantity = 0;
for (size_t i = 0; i < num_orders; ++i) {
auto [price, quantity] = orders[i];
weighted_sum += op(price, quantity);
total_quantity += quantity;
}
return weighted_sum / total_quantity;
}
Here, calculate_weighted_average is a template function that takes a vector of orders, the number of orders to consider, and an operation. The Operation parameter can be a template lambda that defines how to calculate the weighted value.
C++
auto weighted_operation = []<typename PriceType, typename QuantityType>(PriceType price, QuantityType quantity) {
return price * quantity;
};
std::vector<std::pair<int64_t, int64_t>> order_book = {{100, 10}, {101, 20}, {102, 15}};
int64_t average_price = calculate_weighted_average(order_book, 3, weighted_operation);
The compiler will generate a specialized version of calculate_weighted_average for int64_t and the weighted_operation lambda, resulting in highly optimized code.
Advanced Techniques
For even greater performance gains, consider these advanced techniques:
Expression Templates: Combine template lambdas with expression templates to eliminate intermediate computations and generate highly optimized code for complex mathematical expressions.
Compile-Time Branching: Use if constexpr within template lambdas to generate different code paths based on compile-time conditions, eliminating runtime branch prediction overhead.
SIMD Intrinsics: Integrate SIMD intrinsics into template lambdas to perform parallel operations on multiple data elements, further accelerating data-intensive computations.
Custom Allocators: Use custom allocators with template lambdas to reduce memory allocation overhead, which can be critical in HFT applications.
Caveats and Considerations
While template lambdas offer significant performance benefits, they also introduce some complexities:
Increased Compile Times: Template metaprogramming can lead to longer compile times, especially for complex algorithms.3
Code Complexity: Template lambdas can make code more complex and harder to debug.
Binary Size: Generating specialized code for multiple types can increase the size of the executable.
Therefore, it's crucial to use template lambdas judiciously and profile your code to identify performance bottlenecks.
Conclusion
Template lambdas are a powerful tool for optimizing C++ code in HFT applications. By leveraging their ability to generate specialized code at compile time, developers can achieve significant performance gains, reducing latency and increasing throughput. However, it's essential to balance the performance benefits with the increased complexity and compile times. By understanding the fundamentals and applying advanced techniques, HFT developers can harness the full potential of template lambdas to gain a competitive edge in the fast-paced world of high-frequency trading.
Comments