Landing a C++ job in High-Frequency Trading (HFT) is notoriously challenging. These firms seek exceptional programmers who can write ultra-low-latency, high-throughput code.1 Interview processes are rigorous, often involving complex technical questions that probe deep C++ expertise.2 This article explores some of the most difficult C++ questions you might encounter in an HFT interview, categorized for clarity.3
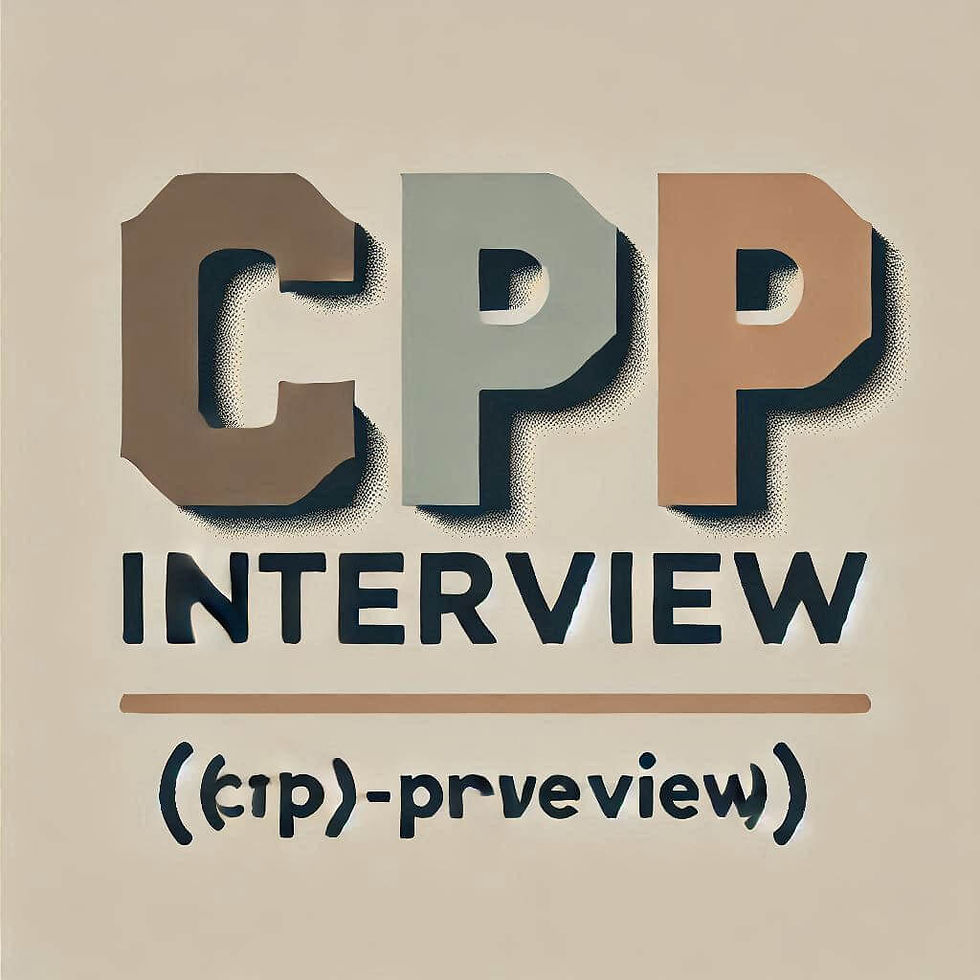
I. Low-Latency Optimization
HFT systems live and die by speed. Microseconds matter, so interviewers will assess your ability to write efficient code. Expect questions like:
Cache-Friendly Code:
"How do you write code that maximizes cache utilization?"
This tests your understanding of CPU caches (L1, L2, L3) and how memory layout affects performance. You should discuss techniques like:
Data Structure Alignment: Aligning data structures to cache line boundaries to avoid cache line splits.
Linear Data Access: Accessing data sequentially to maximize cache hits.
Padding: Adding padding to structures to improve alignment.4
Data Locality: Keeping related data close together in memory.5
Branch Prediction:
"How do you minimize branch mispredictions?"
Branch mispredictions stall the CPU pipeline.6 You should mention:
Branchless Code: Using techniques like conditional moves or bitwise operations to avoid branches altogether.
Likely/Unlikely Attributes: Using compiler hints like __builtin_expect (GCC) or [[likely]]/[[unlikely]] (C++20) to guide branch prediction.
Memory Allocation:
"How would you implement a custom memory allocator for low-latency trading?"
Standard allocators like malloc and new can be slow. You might discuss:
Memory Pools: Pre-allocating large chunks of memory and managing allocation within the pool.7
Free List: Maintaining a list of free blocks for fast allocation and deallocation.
Avoiding Fragmentation: Strategies to minimize memory fragmentation over time.
II. Concurrency and Multithreading
HFT systems are highly concurrent, processing massive amounts of data in parallel. Expect in-depth questions on:
Lock-Free Data Structures:
"Implement a lock-free queue."
This is a classic HFT question. You need to demonstrate understanding of:
Atomic Operations: Using std::atomic for thread-safe data access without locks.
Compare-and-Swap (CAS): A fundamental atomic operation for lock-free programming.
Memory Ordering: Understanding memory models (e.g., sequential consistency, relaxed ordering) and their implications.
Thread Synchronization Primitives:
"Compare and contrast mutexes, semaphores, and condition variables."
You should explain the use cases and performance characteristics of each:
Mutexes: For mutual exclusion, protecting shared resources.8
Semaphores: For controlling access to a limited number of resources.9
Condition Variables: For signaling between threads.10
False Sharing:
"What is false sharing and how do you prevent it?"
False sharing occurs when threads access different data within the same cache line, leading to unnecessary cache invalidations.11 Solutions include:
Padding: Adding padding to data structures to ensure that frequently accessed data resides in separate cache lines.
III. Advanced C++ Features
HFT interviews often delve into advanced C++ concepts:
Template Metaprogramming:
"Write a template metaprogram to calculate the factorial of a number at compile time."
This tests your ability to use templates for compile-time computation.
RAII (Resource Acquisition Is Initialization):
"Explain RAII and give an example of its use in managing network connections."
RAII is crucial for resource management in C++. You should explain how it ensures that resources are automatically released when objects go out of scope.12
Move Semantics and Perfect Forwarding:
"Explain move semantics and how they improve performance."
Move semantics avoid unnecessary copying of objects, especially important for large data structures.13 Perfect forwarding allows you to forward arguments to other functions without losing their original type information.14
IV. System Design
Some interviews may include system design questions:
Order Book Implementation:
"Design an order book for a trading exchange."
This requires knowledge of data structures (e.g., red-black trees, hash tables) and algorithms for efficient order matching.
Handling Market Data:
"How would you design a system to process high-volume market data feeds?"
This involves considerations of throughput, latency, and fault tolerance.
Preparing for the Challenge
To ace an HFT interview, you need:
Deep C++ Knowledge: Master the language, including advanced topics like templates, concurrency, and memory management.
Low-Latency Programming Expertise: Understand techniques for writing efficient code.
System Design Skills: Be able to design and implement complex systems.
Practice: Solve coding problems on platforms like LeetCode and HackerRank.
By thoroughly preparing in these areas, you'll significantly increase your chances of success in the challenging world of HFT interviews.
Comments