Exploring the Best C++ Metaprogramming Libraries for Metafunction Composition
- Bryan Downing
- Jan 6
- 4 min read
Composing Metafunctions: A Deep Dive into Conceptrodon's Architecture
The development of Conceptrodon, a C++ metaprogramming library, has reached a significant milestone. With a readable codebase and comprehensive documentation, the library's core concepts are well-articulated. The introductory material, comprising a README and three key articles – "Hello World," "Vocabulary," and "Functional Nature" – provides a solid foundation for understanding its functionality. This article delves into a crucial aspect of Conceptrodon: the composition of higher-order metafunctions, a mechanism that empowers the library's expressive power and internal transparency.
Conceptrodon's design philosophy centers around uniquely named member templates, each designated to handle specific argument types. This approach ensures transparency within each metafunction, making their internal workings readily understandable. This article will focus on how these member templates facilitate composition.
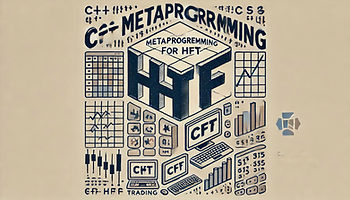
The Foundation: Uniquely Named Member Templates
The core principle of Conceptrodon's architecture lies in the use of distinct member templates to accept different kinds of arguments. This explicit designation clarifies the expected input for each part of a metafunction. Two primary member templates are central to this mechanism: Rail and Page.
Rail: This member template is designed to accept template-head arguments of the form template<auto...>. If a metafunction requires additional arguments that are themselves templates taking auto parameters, it must contain a member template named Rail. The template head of Rail is defined as template<template<auto...> class...>. This specific signature clearly indicates that Rail expects a sequence of template templates, each accepting a variadic number of non-type template parameters.
Page: This member template is responsible for handling additional value arguments. When a metafunction requires extra values as input, it must contain a member template named Page. The template head of Page is template<auto...>. This signature indicates that Page expects a variadic number of non-type template parameters, representing the values passed to the metafunction.
This strict naming convention and type enforcement provide several advantages:
Clarity: The code becomes more readable and understandable. By simply looking at the presence and signature of Rail or Page, one can immediately discern the types of arguments a metafunction expects.
Error Detection: The compiler can enforce type correctness at compile time. If a metafunction is invoked with incorrect argument types, the compiler will generate an error, preventing subtle bugs.
Composition: This structured approach facilitates the composition of higher-order metafunctions, as we will explore in detail.
Composing Metafunctions: The Power of Rail and Page
The true power of Conceptrodon's architecture becomes apparent when composing metafunctions. The explicitly defined Rail and Page member templates act as connection points, allowing complex metafunctions to be built from simpler ones.
Consider a scenario where we want to create a metafunction that transforms a sequence of types using another metafunction. Let's call the transforming metafunction Transformer. Transformer might require a template template as an argument, which it will apply to each type in the input sequence. In Conceptrodon, this would be achieved using the Rail member template.
C++
template <typename T>
struct Identity { using type = T; };
template <typename... Ts>
struct TypeList {};
template <typename List, template <typename> class Transformer>
struct TransformList {
template <template <typename> class... Transformers>
struct Rail {
template <typename... Us>
struct Page {
using type = TypeList<typename Transformer<Us>::type...>;
};
};
};
using TransformedList = TransformList<TypeList<int, double, char>, Identity>::Rail<>::Page<int, double, char>::type; // TypeList<int, double, char>
In this simplified example, TransformList takes a TypeList and a Transformer template. Its
Rail member doesn't actually use the Transformers template pack in this simple case but is present to fulfill the contract of taking a template template. The Page member then applies the Transformer (in this case, Identity) to each type in the original TypeList.
This example demonstrates the basic principle. More complex compositions can be achieved by nesting Rail and Page members or by using them in conjunction with other member templates designed for different argument types.
Higher-Order Composition: Building Complex Transformations
Conceptrodon's architecture allows for higher-order composition, where metafunctions operate on other metafunctions. This is crucial for creating reusable and flexible metaprogramming constructs.
Imagine a metafunction Compose that takes two metafunctions, F and G, and returns a new metafunction that applies G first and then F. This could be implemented by using the Rail member to accept the two metafunctions as template template arguments and then using the Page member to apply them in the correct order.
This higher-order composition enables the creation of powerful abstractions. For instance, one could define metafunctions for common operations like mapping, filtering, and folding over type sequences and then combine them using Compose to create complex transformations.
Benefits of Conceptrodon's Approach
The approach of using uniquely named member templates for argument handling offers several key benefits:
Improved Readability: The code becomes more self-documenting. The presence of Rail and Page clearly indicates the expected argument types.
Enhanced Maintainability: Changes to the internal implementation of a metafunction are less likely to break other parts of the code, as the interface defined by Rail and Page remains consistent.
Increased Composability: Metafunctions can be easily combined to create more complex transformations, promoting code reuse and reducing redundancy.
Compile-Time Safety: The compiler enforces type correctness, catching errors early in the development process.
Conclusion
Conceptrodon's design, with its emphasis on uniquely named member templates like Rail and Page, provides a powerful and elegant mechanism for composing metafunctions. This approach enhances code readability, maintainability, and composability while ensuring compile-time safety. By understanding how these member templates work, developers can leverage Conceptrodon's full potential to create complex and efficient metaprogramming solutions. This structured approach to composition is a key differentiator for Conceptrodon, enabling the construction of sophisticated compile-time algorithms in a clear and maintainable way.
Comments