Implementing a C++ Quant Trading System: Step-by-Step Guide to Building a Backtester
- Bryan Downing
- Feb 13
- 4 min read
Backtesting is the cornerstone of quantitative trading. It's the process of simulating a trading strategy on historical data to assess its performance, identify potential weaknesses, and ultimately determine its viability for live trading. A robust backtesting framework is essential for accurate and reliable results in quant trading. This article provides a comprehensive guide to building such a framework in C++, a language well-suited for the performance demands of quantitative finance.
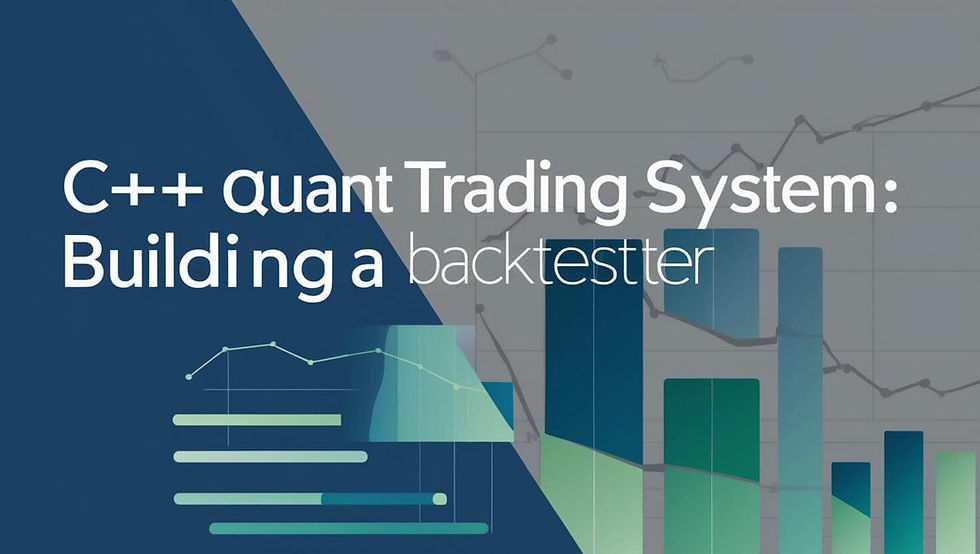
Introduction: The Power of Backtesting in Quant Trading
Backtesting serves several critical purposes:
Strategy Validation: It allows you to test the effectiveness of a trading strategy before risking real capital. By simulating the strategy on historical data, you can get a sense of how it would have performed in the past.
Risk Management: Backtesting helps identify potential risks associated with a trading strategy. You can analyze historical drawdowns, maximum losses, and other risk metrics to understand the potential downsides.
Parameter Optimization: Backtesting enables you to optimize the parameters of a trading strategy. By testing different parameter combinations, you can find the settings that maximize performance on historical data.
A well-designed backtesting framework is crucial for obtaining reliable results. It must accurately simulate market conditions, handle various data types, and provide comprehensive performance metrics.
C++ is a popular choice for quantitative finance due to its performance, control, and extensive libraries. Its ability to handle complex calculations and large datasets efficiently makes it ideal for building robust backtesting systems.
C++ Fundamentals for Quant Trading
Before diving into backtesting, let's review some essential C++ concepts:
Data Structures: Understanding data structures like vectors, maps, and queues is crucial for storing and manipulating market data.
Algorithms: Knowledge of algorithms for searching, sorting, and other operations is essential for implementing trading logic.
Object-Oriented Programming (OOP): OOP principles like encapsulation, inheritance, and polymorphism can help you design a modular and maintainable backtesting framework.
Here's an example of a basic trading data class:
C++
#include <iostream>
#include <vector>
struct MarketData {
long timestamp;
double open;
double high;
double low;
double close;
long volume;
};
std::vector<MarketData> historicalData; // Store historical market data
Efficiency is paramount in quantitative trading. Using appropriate data structures and algorithms, minimizing memory allocations, and leveraging techniques like vectorization are crucial for optimizing performance.
Building a Backtester Framework
A modular backtester framework should have clear separation of concerns:
Data Loading: Responsible for loading historical market data from various sources (e.g., CSV files, databases, APIs).
Order Execution: Simulates the execution of trading orders, taking into account factors like slippage and market impact.
Position Management: Tracks open positions, calculates profit and loss (P&L), and manages risk.
Strategy Logic: Implements the trading strategy, generating buy and sell signals based on market data.
Performance Metrics: Calculates key performance metrics like Sharpe ratio, maximum drawdown, and return on investment (ROI).
Here's a simplified example of order execution:
C++
struct Order {
enum class OrderType { BUY, SELL };
std::string symbol;
OrderType type;
int quantity;
double price;
};
void executeOrder(const Order& order) {
// ... (Simulate order execution logic, considering slippage, etc.) ...
if (order.type == Order::OrderType::BUY) {
// ... (Update position and calculate P&L) ...
} else if (order.type == Order::OrderType::SELL) {
// ... (Update position and calculate P&L) ...
}
}
The backtester should be configurable with different market data, timeframes, trading parameters, and strategies.
Backtesting a Simple Strategy
Let's define a simple moving average crossover strategy:
Buy Signal: When the short-term moving average crosses above the long-term moving average.
Sell Signal: When the short-term moving average crosses below the long-term moving average.
Implementing this strategy within the backtester involves iterating through the historical data, calculating the moving averages, and generating buy/sell signals based on the crossover conditions.
After running the backtest, analyze the results to assess the strategy's performance. Look at metrics like:
Profitability: Overall return, annualized return.
Risk: Maximum drawdown, volatility.
Sharpe Ratio: Risk-adjusted return.
Advanced Backtesting Techniques
Advanced backtesting techniques can provide a more comprehensive evaluation of a trading strategy:
Walk-Forward Analysis: This technique involves dividing the historical data into in-sample and out-of-sample periods. The strategy is optimized on the in-sample period and then tested on the out-of-sample period. This process is repeated, moving the in-sample and out-of-sample periods forward.
Monte Carlo Simulations: These simulations involve running the backtest multiple times with slightly different market data or parameters. This helps assess the robustness of the strategy and its sensitivity to small changes in market conditions.
Stress Testing: Stress testing involves subjecting the strategy to extreme market conditions to evaluate its performance under adverse scenarios.
C++'s performance makes it well-suited for implementing these computationally intensive techniques. Furthermore, libraries like those for machine learning or genetic optimization can be integrated into your C++ backtester to automate parameter optimization.
Conclusion
Building a robust backtester in C++ is a crucial step in developing a successful quant trading system. A well-designed backtester allows you to thoroughly evaluate trading strategies, manage risk effectively, and optimize performance. By combining a solid understanding of C++ with sound software engineering principles, you can create a powerful tool for navigating the complexities of the financial markets.
Comments