How to Incorporate Real-Time HFT System in Python for Quant Developers?
- Bryan Downing
- Feb 13
- 4 min read
High-frequency trading (HFT) represents the cutting edge of financial markets, characterized by its lightning-fast speed, sophisticated automation, and significant market impact.1 HFT firms leverage complex algorithms to execute trades in milliseconds, capitalizing on fleeting market opportunities.2 Building such a system is a challenging endeavor, requiring expertise in data management, algorithm development, and low-latency execution.3 Python, with its robust libraries and mature ecosystem, has emerged as a powerful tool for developing HFT systems.4 This article provides a comprehensive guide to building a real-time HFT system using Python for an aspiring quant developer.
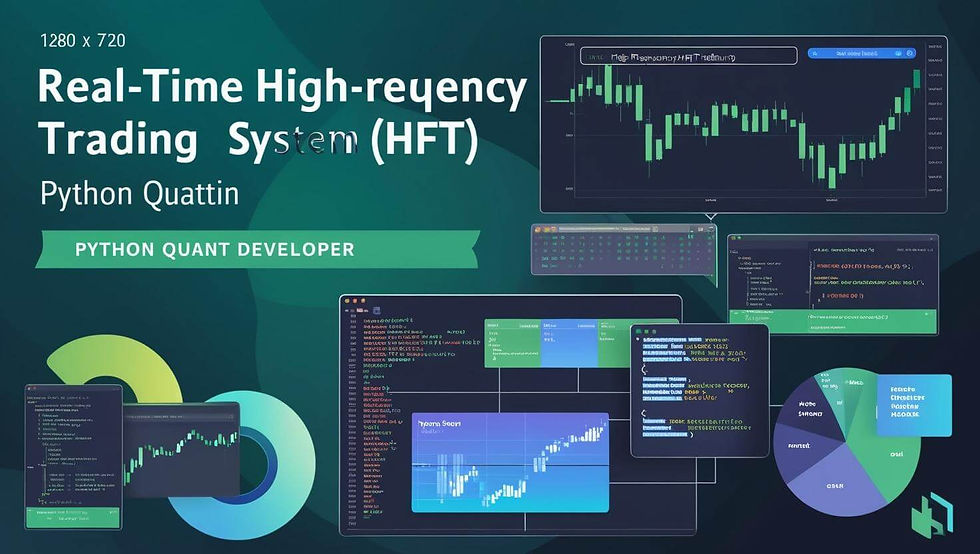
What is HFT & Why Python?
HFT involves executing a large number of orders at extremely high speeds.5 The core principles are:
Speed: Milliseconds matter.6 HFT systems strive to minimize latency and execute trades as quickly as possible.7
Automation: Trades are executed automatically by algorithms, eliminating human intervention.8
Market Impact: HFT can significantly impact market liquidity and price discovery.9
HFT faces several challenges:
Latency: Minimizing delays in data acquisition, processing, and order execution is crucial.10
Data Management: Handling massive amounts of real-time market data efficiently is essential.11
Complex Algorithms: Developing profitable HFT strategies requires sophisticated algorithms and rigorous testing.12
Python offers several advantages for HFT development:
Robust Libraries: Libraries like Pandas, NumPy, and Scikit-learn provide powerful tools for data manipulation, numerical computation, and machine learning.13
Mature Ecosystem: Python's large and active community provides ample support and resources.14
Ease of Use: Python's syntax is relatively easy to learn, making it accessible to developers with varying levels of experience.15
Project Setup & Libraries
Setting up the development environment is the first step:
Install Python: Download and install the latest version of Python.
IDE: Choose an Integrated Development Environment (IDE) like PyCharm or VS Code.
Libraries: Install essential libraries using pip: pip install pandas numpy matplotlib yfinance (yfinance for fetching historical data - you'll need a real-time data source for live trading).
Key libraries:
Pandas: For data manipulation and analysis.16
NumPy: For numerical computations and array operations.17
Matplotlib: For data visualization.18
yfinance: For historical market data (for backtesting - not live trading).
A well-organized project structure is crucial. Consider a structure like this:
hft_system/
├── data/ # Store market data
├── strategies/ # Implement trading strategies
├── utils/ # Helper functions
├── backtester.py # Backtesting module
├── live_trader.py # Live trading module
└── main.py # Main script
Data Acquisition & Preprocessing
For real-time data, you'll need a suitable provider. Options include:
Broker APIs: Many brokers offer APIs for accessing real-time market data.19
Financial Data Providers: Companies like Bloomberg or Refinitiv provide comprehensive market data feeds (often at a cost).20
Web Scraping (Limited): Web scraping might be an option for some data, but it's often unreliable and not suitable for true HFT due to latency.
Data preprocessing is essential:
Data Cleaning: Handle missing values, outliers, and inconsistencies in the data.
Normalization: Normalize or standardize the data to improve the performance of your algorithms.
Feature Engineering: Create new features from the raw data that might be useful for your trading strategies. Examples include moving averages, relative strength index (RSI), or volatility measures.
Building the HFT Algorithm
Backtesting is crucial for developing and evaluating trading strategies:
Historical Data: Use historical market data (e.g., from yfinance) to simulate trading.
Strategy Development: Implement your HFT strategy using Python. A simple example could be a moving average crossover strategy or an arbitrage strategy.
Backtesting Framework: Develop a backtesting framework to simulate trades, calculate performance metrics (e.g., Sharpe ratio, maximum drawdown), and analyze the results.
Example (simplified moving average crossover):
Python
import pandas as pd
import yfinance as yf
# ... (data loading and preprocessing) ...
df['SMA_50'] = df['Close'].rolling(window=50).mean()
df['SMA_200'] = df['Close'].rolling(window=200).mean()
df['Signal'] = 0
df['Signal'][df['SMA_50'] > df['SMA_200']] = 1
df['Signal'][df['SMA_50'] < df['SMA_200']] = -1
# ... (backtesting logic and performance evaluation) ...
Optimization involves tuning the parameters of your strategy to improve performance.21
Deployment & Considerations
Deploying an HFT system requires careful planning:
Hardware: You'll need powerful servers with low latency connections to the exchanges. Colocation (placing your servers close to the exchange's servers) is often used in HFT.22
Cloud Computing: Cloud platforms can provide scalability and flexibility, but latency can be a concern.23
Dedicated Servers: For maximum performance, dedicated servers are often preferred.
Monitoring and maintenance are essential:
Real-time Monitoring: Track the performance of your system in real-time.
Error Handling: Implement robust error handling to prevent unexpected issues.
System Updates: Keep your system up-to-date with the latest market data and software.
Ethical and regulatory considerations are paramount:
Market Manipulation: Avoid any actions that could be construed as market manipulation.
Order Book Manipulation: Be aware of regulations regarding order book manipulation.
Legal Compliance: Ensure that your trading activities comply with all applicable laws and regulations.
Building a real-time HFT system is a complex undertaking. It requires a deep understanding of financial markets, programming, data analysis, and risk management. This article provides a starting point for your HFT journey. Remember to thoroughly research and test your strategies before deploying them in live trading.
Kommentit